前回は画像提示した4択クイズを作成することをしました。
もちろんchatGPTに丸投げしています。笑
今回はCSVファイルで作成したクイズを表出するプログラムを作成しました。
ランダムにクイズを出題して、回答ボタンを押し正解すると加算されるという具合になります。
今回の場合は段階を経て修正した過程を記事にしました。
雛形のCSVファイルを用いたプログラムはすでにChatGPTを使って作成しています。
初期段階
初期段階は以下のコードになります。
import tkinter as tk
import random
import csv
current_question_index = -1
question_label = None
image = None # 画像オブジェクト
# CSVファイルから問題と画像のリストを読み込む関数
def load_quiz_data(file_path):
quiz_data = []
with open(file_path, “r”, encoding=”utf-8″) as file:
reader = csv.reader(file)
next(reader) # 題名行をスキップ
for row in reader:
question = row[0]
image_path = row[1]
choices = row[2:]
quiz_data.append((question, image_path, choices))
return quiz_data
# クイズ画面を作成する関数
def create_quiz(file_path):
global current_question_index, question_label, image
# ウィンドウを作成
window = tk.Tk()
window.title(“Quiz”)
# 問題と画像のリストを読み込む
quiz_data = load_quiz_data(file_path)
# 問題の順番をランダムにする
random.shuffle(quiz_data)
# ランダムに問題を選択
current_question_index = 0
question, image_path, choices = quiz_data[current_question_index]
# 画像を表示するキャンバスを作成
canvas = tk.Canvas(window, width=500, height=500)
canvas.pack()
image = tk.PhotoImage(file=image_path)
image_id = canvas.create_image(250, 250, image=image)
# 問題を表示
question_label = tk.Label(window, text=question)
question_label.pack()
# 回答選択肢を表示するフレームを作成
answer_frame = tk.Frame(window) # 追加: 回答選択肢フレームを作成
answer_frame.pack()
correct_choice = choices[0] # 正解を取得
incorrect_choices = choices[1:] # 不正解の選択肢を取得
# 正解と不正解の選択肢をシャッフルして結合
answer_choices = random.sample(incorrect_choices, len(incorrect_choices))
answer_choices.append(correct_choice)
random.shuffle(answer_choices)
answer_buttons = []
for answer_choice in answer_choices:
answer_button = tk.Button(answer_frame, text=answer_choice, width=25)
answer_button.pack(pady=5)
answer_buttons.append(answer_button)
def show_next_question():
global current_question_index, question_label, image
current_question_index += 1
if current_question_index < len(quiz_data):
question, image_path, choices = quiz_data[current_question_index]
question_label.config(text=question)
image = tk.PhotoImage(file=image_path)
canvas.itemconfig(image_id, image=image)
correct_choice = choices[0] # 正解を取得
incorrect_choices = choices[1:] # 不正解の選択肢を取得
answer_choices = random.sample(incorrect_choices, len(incorrect_choices))
answer_choices.append(correct_choice)
random.shuffle(answer_choices)
for i, choice in enumerate(answer_choices):
answer_buttons[i].config(text=choice)
else:
window.destroy()
for answer_button in answer_buttons:
answer_button.config(command=show_next_question)
window.mainloop()
# クイズを実行する
create_quiz(“image/quiz_data2.csv”)
そうすると、こんな画像ですね。
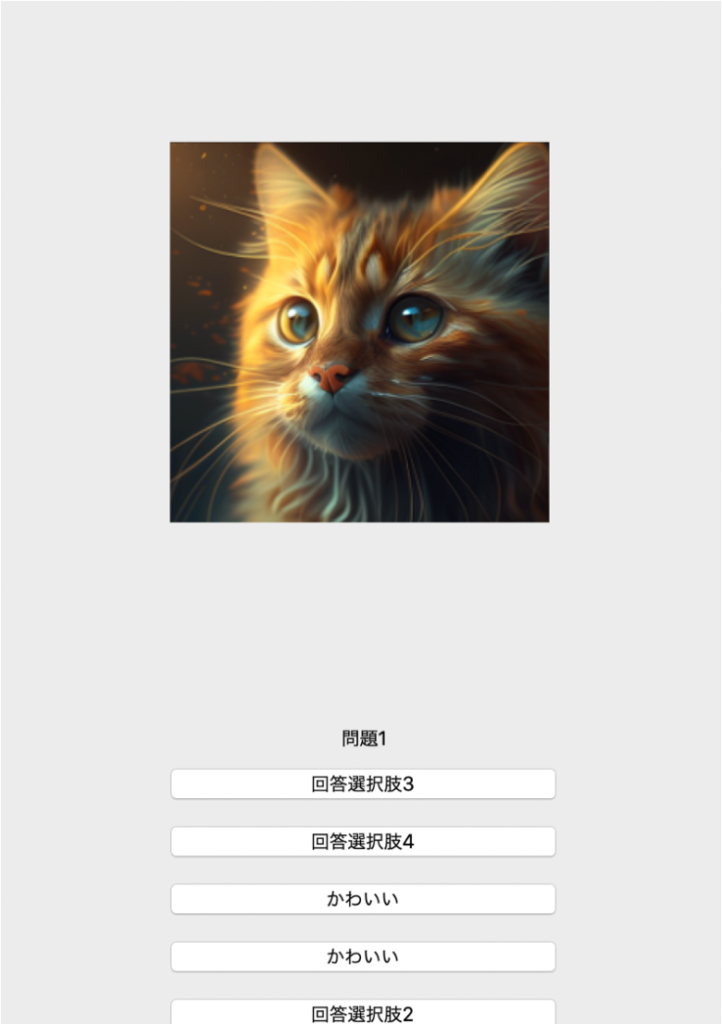
これだと、csvファイルで定義していた答えまでもが選択肢になっております。
これは修正してもらいましょう。
ChatGPTにCSVファイルの答えの選択肢は提示しないように指示を書きました。
選択肢を提示しないように
import tkinter as tk
import csv
import random
current_question_index = -1
question_label = None
image = None # 画像オブジェクト
answer_buttons = []
quiz_data = []
score = 0 # スコアの初期値
# CSVファイルから問題と画像のリストを読み込む関数
def load_quiz_data(file_path):
quiz_data = []
with open(file_path, “r”, encoding=”utf-8″) as file:
reader = csv.reader(file)
next(reader) # 題名行をスキップ
for row in reader:
question = row[0]
image_path = row[1]
choices = row[2:-1] # 最後の列を除く回答選択肢
correct_answer = row[-1] # 正解の選択肢
quiz_data.append((question, image_path, choices, correct_answer))
return quiz_data
# クイズ画面を作成する関数
def create_quiz(file_path):
global current_question_index, question_label, image, answer_buttons, quiz_data, score
# ウィンドウを作成
window = tk.Tk()
window.title(“Quiz”)
# 問題と画像のリストを読み込む
quiz_data = load_quiz_data(file_path)
# 問題の順番をランダムにする
random.shuffle(quiz_data)
# ランダムに問題を選択
current_question_index = 0
question, image_path, choices, correct_answer = quiz_data[current_question_index]
# 画像を表示するキャンバスを作成
canvas = tk.Canvas(window, width=500, height=500)
canvas.pack()
image = tk.PhotoImage(file=image_path)
image_id = canvas.create_image(250, 250, image=image)
# 問題を表示
question_label = tk.Label(window, text=question)
question_label.pack()
# 回答選択肢を表示するフレームを作成
answer_frame = tk.Frame(window) # 追加: 回答選択肢フレームを作成
answer_frame.pack()
def check_answer(selected_choice):
global score
if selected_choice == correct_answer:
score += 1
show_next_question()
def show_next_question():
global current_question_index, question_label, image, answer_buttons
current_question_index += 1
if current_question_index < len(quiz_data):
question, image_path, choices, correct_answer = quiz_data[current_question_index]
question_label.config(text=question)
image = tk.PhotoImage(file=image_path)
canvas.itemconfig(image_id, image=image)
for i, answer_choice in enumerate(choices):
answer_buttons[i].config(text=answer_choice, command=lambda choice=answer_choice: check_answer(choice))
else:
show_result()
def show_result():
result_text = f”スコア: {score}/{len(quiz_data)}”
result_label = tk.Label(window, text=result_text)
result_label.pack()
for answer_choice in choices:
answer_button = tk.Button
answer_button = tk.Button(answer_frame, text=answer_choice, width=25, command=lambdachoice=answer_choice: check_answer(choice))
answer_button.pack(pady=5)
answer_buttons.append(answer_button)
window.mainloop()
# クイズを実行する
create_quiz(“image/quiz_data2.csv”)
これで、ほぼ完成です。
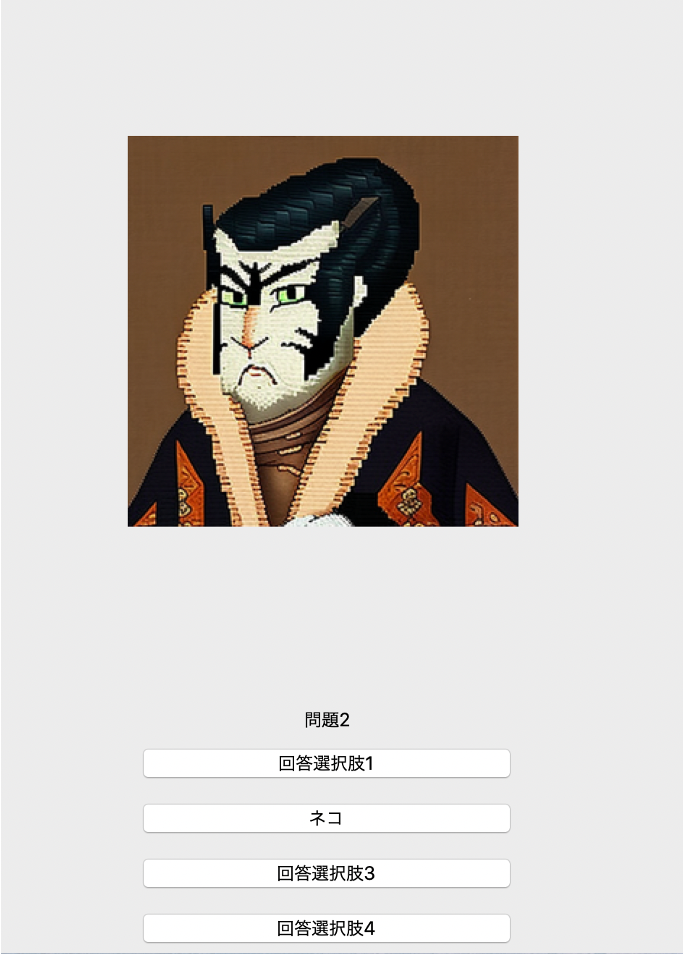
これはミッドジャーニーのbrendで猫と歌舞伎の浮世絵を合成して作成した画像です。
これだと、正解を押しても点数がちゃんと加算されていなかったので、もう一回加算されるようにお願いしました。
先ほどのコードをコピペして貼り付けて、最後に追加の質問をしています。
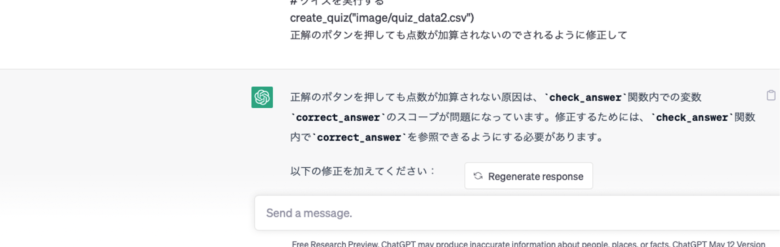
これだけではエラーになったので、再度エラー項目をコピペして質問しました。
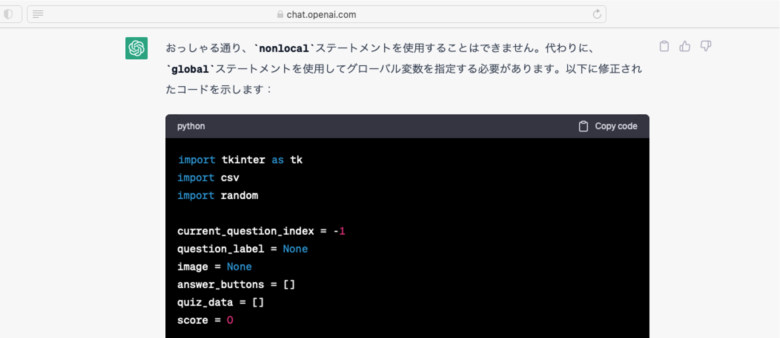
するとlocalとかglobalとかうんたらと記載してくれています。
正直よくわかりません。笑
でも修正してみました。
加算されるようにする
import tkinter as tk
import csv
import random
current_question_index = -1
question_label = None
image = None
answer_buttons = []
quiz_data = []
score = 0
def load_quiz_data(file_path):
quiz_data = []
with open(file_path, “r”, encoding=”utf-8″) as file:
reader = csv.reader(file)
next(reader)
for row in reader:
question = row[0]
image_path = row[1]
choices = row[2:-1]
correct_answer = row[-1]
quiz_data.append((question, image_path, choices, correct_answer))
return quiz_data
def create_quiz(file_path):
global current_question_index, question_label, image, answer_buttons, quiz_data, score
window = tk.Tk()
window.title(“Quiz”)
quiz_data = load_quiz_data(file_path)
random.shuffle(quiz_data)
current_question_index = 0
question, image_path, choices, correct_answer = quiz_data[current_question_index]
canvas = tk.Canvas(window, width=500, height=500)
canvas.pack()
image = tk.PhotoImage(file=image_path)
image_id = canvas.create_image(250, 250, image=image)
question_label = tk.Label(window, text=question)
question_label.pack()
answer_frame = tk.Frame(window)
answer_frame.pack()
def check_answer(selected_choice):
global score, current_question_index
question, image_path, choices, correct_answer = quiz_data[current_question_index]
if selected_choice == correct_answer:
score += 1
show_next_question()
def show_next_question():
global current_question_index, question_label, image, answer_buttons
current_question_index += 1
if current_question_index < len(quiz_data):
question, image_path, choices, correct_answer = quiz_data[current_question_index]
question_label.config(text=question)
image = tk.PhotoImage(file=image_path)
canvas.itemconfig(image_id, image=image)
for i, answer_choice in enumerate(choices):
answer_buttons[i].config(text=answer_choice, command=lambda choice=answer_choice: check_answer(choice))
else:
show_result()
def show_result():
result_text = f”スコア: {score}/{len(quiz_data)}”
result_label = tk.Label(window, text=result_text)
result_label.pack()
for answer_choice in choices:
answer_button = tk.Button(answer_frame, text=answer_choice, width=25, command=lambdachoice=answer_choice: check_answer(choice))
answer_button.pack(pady=5)
answer_buttons.append(answer_button)
window.mainloop()
# クイズを実行する
create_quiz(“image/quiz_data2.csv”)
これを入力すると、
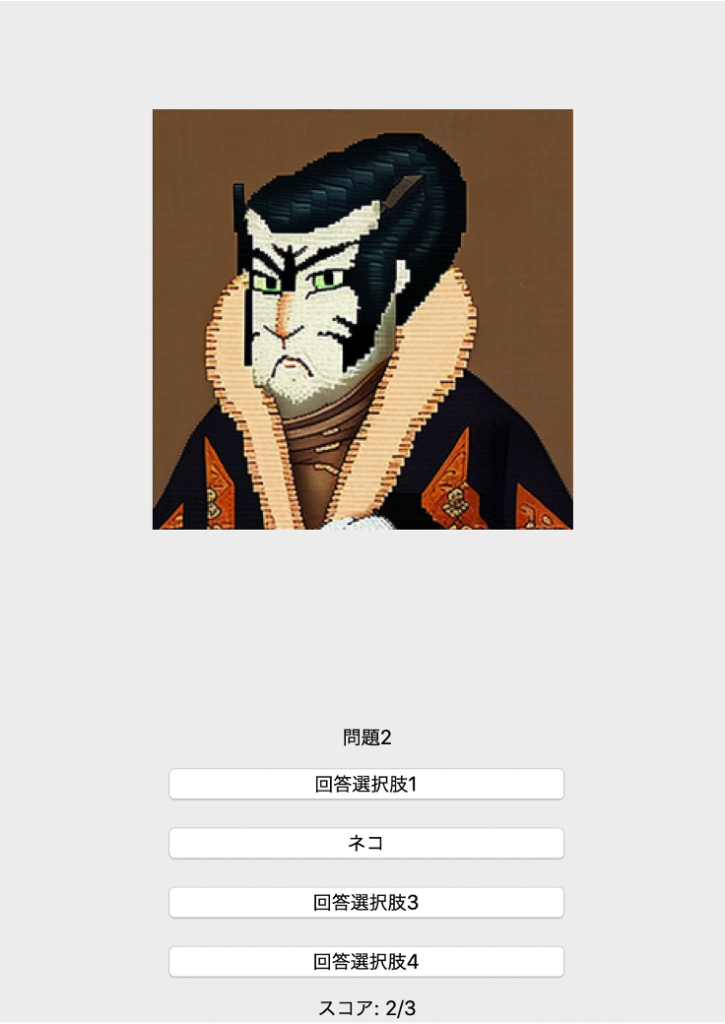
やっとここまでたどり着きました。
まとめ
CSVファイルで作成したクイズを表出するプログラムを作成しました。
自力ではほぼ不可能でしたが、ChatGPTを用いることで作成できました。
ここにいくまでも何度も修正を繰り返しています。
でも修正箇所をchatGPTに入力したら、大概なんとかなります。
ただ修正する時の指示の的確さも必要と感じました。
もっとコードの知識があれば、修正箇所だけわかるのですが、わからないのでどうしても全体の修正になってしまいます。
それが時間がかかる要因ですね。
もう少し改変してクイズアプリを作成していきたいと思っています。
以上参考になれば幸いです。
コメント