Chat GPTは、OpenAIが開発した自然言語処理に特化したAIモデルですね。
Chat GPTは様々な分野での課題解決に役立つことが期待されております。
文書の自動要約、翻訳、質問応答、文章生成などにも応用されています。
文章作成も面白いのですが、プログラムのコードを記述してくれるのは画期的と思います。
以前も簡単なカレンダーを作成しました。
すごい流行しています。
しかし私は医療界にいる影響か、あんまり話題にしている人を見かけません。
環境の問題かもしれませんが。
本題ですが、
PythonのGUIアプリケーションを作成することは、非常に面白いです。
何回か挫折したましたが笑
将来的により高度なGUIアプリケーションを作りたいと考えています。
そのために、まずは簡単なクイズアプリを作ってみました。
正確にいうとChat GPTがですけど笑
Chat GPTがコードを記述してくれますが、クイズアプリを作ることで、PythonのGUIプログラミングの基礎は学ぶことができると思います。
今回はTkinterというPythonのGUIライブラリを使用してクイズアプリを作成しました。
TkinterはPythonに標準で搭載されているGUIライブラリであり、初心者でも簡単に使うことができます。
Tkinterは豊富なウィジェットのライブラリがあるため、見た目や機能性に優れたGUIを簡単に作成することができます。
4択のクイズアプリを作って、パイソン のGUIで
こんな感じで記載するだけ、コードを教えてくれます。
教えてくれたコードはこれです。
from tkinter import *
#正解の回答
answer = “スーパーマリオブラザーズ”
#ウィンドウの作成
root = Tk()
root.title(“4択クイズアプリ”)
#ウィンドウの大きさを設定
root.geometry(“600×400”)
#クイズの質問ラベル
question_label = Label(root, text=”次のうち、最初にリリースされたゲームはどれですか?”, font=(“Arial”, 16))
question_label.pack(pady=20)
#選択肢ボタン
button1 = Button(root, text=”スーパーマリオブラザーズ”, font=(“Arial”, 14), width=25)
button2 = Button(root, text=”ドンキーコング”, font=(“Arial”, 14), width=25)
button3 = Button(root, text=”ゼルダの伝説”, font=(“Arial”, 14), width=25)
button4 = Button(root, text=”メトロイド”, font=(“Arial”, 14), width=25)
#ボタンをウィンドウに配置
button1.pack(pady=10)
button2.pack(pady=10)
button3.pack(pady=10)
button4.pack(pady=10)
#正解判定関数
def check_answer():
if button1[“text”] == answer:
result_label.config(text=”正解!”)
else:
result_label.config(text=”不正解!”)
#結果ラベル
result_label = Label(root, text=””, font=(“Arial”, 16))
result_label.pack(pady=20)
#ボタンに正解判定関数を割り当てる
button1.config(command=check_answer)
button2.config(command=check_answer)
button3.config(command=check_answer)
button4.config(command=check_answer)
#次の質問ボタン
def next_question():
question_label.config(text=”次の質問です。”)
button1.config(text=”回答1″)
button2.config(text=”回答2″)
button3.config(text=”回答3″)
button4.config(text=”回答4″)
result_label.config(text=””)
next_button = Button(root, text=”次の質問”, font=(“Arial”, 14), command=next_question)
next_button.pack(pady=20)
root.mainloop()
これを実行すると、このような画面ができています。
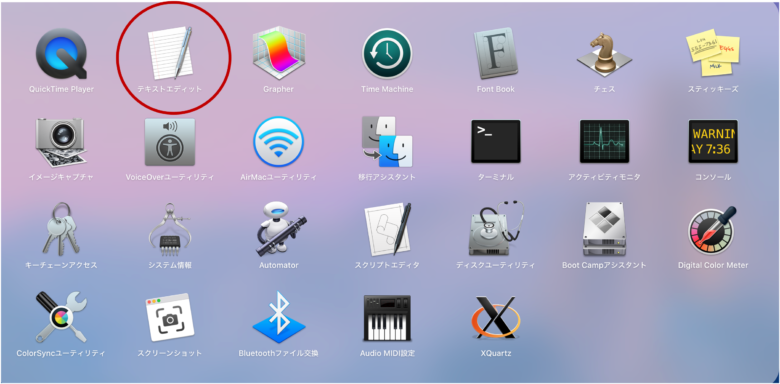
これのボタンを押すと、正解か不正解が出る仕様です。
しかし、どの選択肢も正解になっていました。
ChatGPTは結構プログラムのミスは起こします。
何回か修正をお願いしてみる
その結果新しいコードをくれます。
間違っていることもあるので注意しましょう。
from tkinter import *
# 正解の回答
answer = “スーパーマリオブラザーズ”
# ウィンドウの作成
root = Tk()
root.title(“4択クイズアプリ”)
# ウィンドウの大きさを設定
root.geometry(“600×400”)
# クイズの質問ラベル
question_label = Label(root, text=”次のうち、最初にリリースされたゲームはどれですか?”, font=(“Arial”, 16))
question_label.pack(pady=20)
# 選択肢ボタン
button1 = Button(root, text=”ドンキーコング”, font=(“Arial”, 14), width=25)
button2 = Button(root, text=”スーパーマリオブラザーズ”, font=(“Arial”, 14), width=25)
button3 = Button(root, text=”ゼルダの伝説”, font=(“Arial”, 14), width=25)
button4 = Button(root, text=”メトロイド”, font=(“Arial”, 14), width=25)
# ボタンをウィンドウに配置
button1.pack(pady=10)
button2.pack(pady=10)
button3.pack(pady=10)
button4.pack(pady=10)
# 正解判定関数
def check_answer(selected_answer):
if selected_answer == answer:
result_label.config(text=”正解!”)
else:
result_label.config(text=”不正解!”)
# 結果ラベル
result_label = Label(root, text=””, font=(“Arial”, 16))
result_label.pack(pady=20)
# ボタンに正解判定関数を割り当てる
button1.config(command=lambda: check_answer(button1[“text”]))
button2.config(command=lambda: check_answer(button2[“text”]))
button3.config(command=lambda: check_answer(button3[“text”]))
button4.config(command=lambda: check_answer(button4[“text”]))
# 次の質問ボタン
def next_question():
question_label.config(text=”次の質問です。”)
button1.config(text=”ドンキーコング”)
button2.config(text=”スーパーマリオブラザーズ”)
button3.config(text=”ゼルダの伝説”)
button4.config(text=”メトロイド”)
result_label.config(text=””)
next_button = Button(root, text=”次の質問”, font=(“Arial”, 14), command=next_question)
next_button.pack(pady=20)
root.mainloop()
出来上がりは、
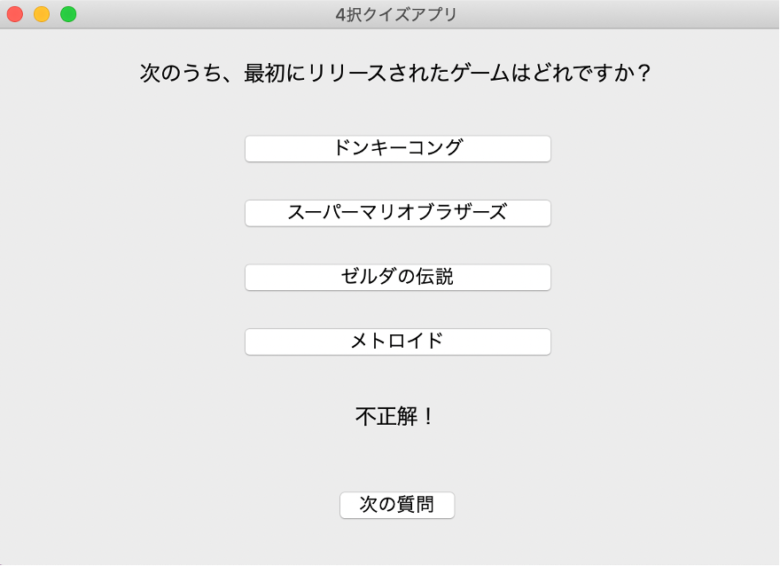
今度はメトロイドを選択すると、ちゃんと不正解になりましたね。
次の質問を押しても質問は変わりませんが、そこら辺は修正していません。
PythonのGUIでクイズゲーム作ってくれ
確かこんな感じに入力した気がします。また別の仕様のプログラムを書いてくれました。
import tkinter as tk
import random
# 問題と答えのリスト
questions = [“What is the capital of Japan?”, “What is the smallest country in the world?”,
“What is the highest mountain in the world?”, “What is the currency of Italy?”,
“What is the largest country by area?”]
answers = [“Tokyo”, “Vatican City”, “Mount Everest”, “Euro”, “Russia”]
# 問題数を取得する
num_questions = len(questions)
# 初期スコア
score = 0
# 問題をシャッフルする
question_order = list(range(num_questions))
random.shuffle(question_order)
# 次の問題を設定する関数
def next_question():
global current_question
if current_question < num_questions:
# 問題文を更新する
question_text.set(questions[question_order[current_question]])
# ボタンのテキストを更新する
answer_button.configure(text=””)
else:
# 全ての問題が終了した場合は結果を表示する
question_text.set(“Your final score is ” + str(score) + “/” + str(num_questions))
# ボタンを無効化する
answer_button.configure(state=”disabled”)
# 答えを確認する関数
def check_answer():
global current_question, score
if current_question < num_questions:
# 正解の場合はスコアを加算する
if answer_text.get().strip().lower() == answers[question_order[current_question]].lower():
score += 1
# 次の問題に進む
current_question += 1
next_question()
# ウィンドウを作成する
root = tk.Tk()
root.title(“Quiz Game”)
# 問題文を表示するラベル
question_text = tk.StringVar()
question_label = tk.Label(root, textvariable=question_text, font=(“Helvetica”, 14), wraplength=500, justify=”center”)
question_label.pack(pady=20)
# 回答欄を作成する
answer_text = tk.StringVar()
answer_entry = tk.Entry(root, textvariable=answer_text, font=(“Helvetica”, 12), width=40)
answer_entry.pack(pady=10)
# 回答ボタンを作成する
answer_button = tk.Button(root, text=”Answer”, font=(“Helvetica”, 12), width=10, command=check_answer)
answer_button.pack(pady=10)
# ゲーム開始
current_question = 0
next_question()
# ウィンドウを表示する
root.mainloop()
出来上がりは、こんな感じです。
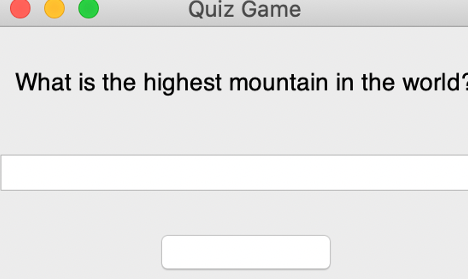
下のボタンを押すと次の問題に行きます。
このクイズは記述式です。
答えを上の空欄に記載しボタンを押すと、正解かどうかカウントしてくれます。
問題が終われば、最後に点数を教えてくれます。
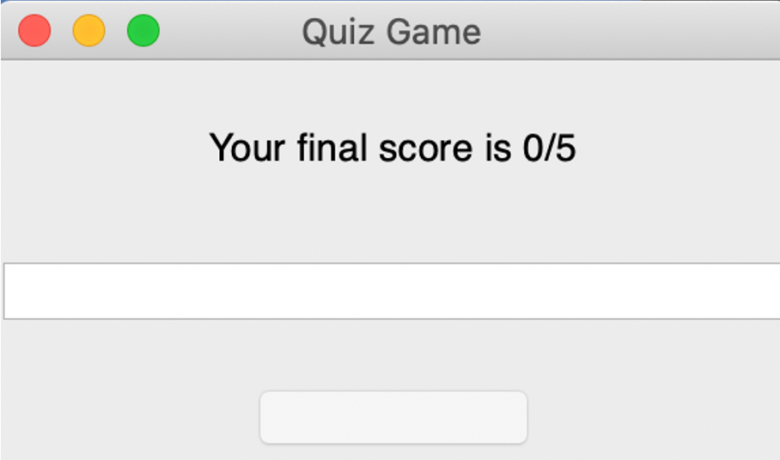
まとめ
Chat GPTを用いて、簡単なクイズアプリを作ってみました。
Chat GPT ではPythonを用いたクイズアプリの開発を素人でも比較的簡単に行うことができます。
Chat GPTがでる以前に、独学でpythonで似たようなアプリを作っていましたが、時間は遥かにかかっていました。
明らかに楽です。
GUIアプリケーションを作ることで、そのアプリケーションがどのように動作するかを直感的に理解することができます
コードの勉強だけしてても、正直つまらないので、こういう風に視覚的に何か出来上がった方が気分も良いと思います。
今度はCSVファイルを使ったクイズ問題を作成したいと思います。
以上参考になれば幸いです。
コメント